mozilla-sync.cpp File Reference
Detailed Description
The mozilla-sync plugin itself.
#include <stdlib.h>
#include <stdio.h>
#include <string.h>
#include <opensync/opensync.h>
#include <opensync/opensync-format.h>
#include <opensync/opensync-plugin.h>
#include <opensync/opensync-version.h>
#include <opensync/opensync-context.h>
#include <opensync/opensync-data.h>
#include <opensync/opensync-helper.h>
#include <opensync/opensync-merger.h>
#include <opensync/opensync-xmlformat.h>
#include "mozilla-xpcom.h"
#include "thunderbird-addressbook.h"
#include "mozilla-calendar.h"
#include "thunderbird-card.h"
#include "calendar-event.h"
#include "mozilla-sync.h"

Classes | |
struct | _CapDef |
Defines | |
#define | ERR_PRINTF(args...) fprintf(stderr, args); |
#define | GFREE(p) { if (p) g_free(p); } |
#define | LOG(level, message...) |
#define | LOG_ENTRY(level, message...) |
#define | LOG_EXIT(level, message...) |
#define | LOG_EXIT_ERROR_OSYNC(level, ppOSyncError) |
#define | LOG_EXIT_ERROR_OSYNC_SZ(level, ppOSyncError, message) |
#define | LOG_EXIT_ERROR_SZ(level, message) |
#define | LOG_EXIT_REPORT_ERROR_MSG(level, context, message...) |
#define | LOG_EXIT_REPORT_ERROR_OSYNC(level, context, pError) |
#define | LOG_EXIT_REPORT_ERROR_SZ(level, context, message) |
#define | LOG_SENSITIVE(level, message...) |
#define | LOGLEVEL 10000 |
Typedefs | |
typedef struct _CapDef | CapDef |
Functions | |
void | addCapabilityEvent (void *voidSz, void *voidPCapDef) |
void | freeMozillaDatabase (void *voidOSyncMozillaDatabase, void *notused) |
osync_bool | get_sync_info (OSyncPluginEnv *pOSyncPluginEnv, OSyncError **ppOSyncError) |
register the mozilla-sync plugin | |
int | get_version (void) |
return version number of plugin | |
void | logFunction (int level, bool sensitive, const char *szFile, int iLine, const char *szFunc, const char *szFormat,...) |
static gboolean | mozilla_sync_commit_addressbook_change (ThunderbirdAddressbook *pThunderbirdAddressbook, OSyncChangeType changeType, const char *szKey, const char *szXML, OSyncHashTable *pHT, OSyncContext *pOSyncContext, OSyncChange *pChange) |
Commit change into thunderbird. | |
static gboolean | mozilla_sync_commit_calendar_change (MozillaCalendar *pCalendar, OSyncChangeType changeType, const char *szKey, const char *szXML, OSyncHashTable *pHT, OSyncContext *pOSyncContext, OSyncChange *pChange) |
Commit change into Mozilla calendar. | |
static void | mozilla_sync_commit_change (void *data, OSyncPluginInfo *pOSyncPluginInfo, OSyncContext *pOSyncContext, OSyncChange *pChange) |
Commit change. | |
static void | mozilla_sync_connect (void *data, OSyncPluginInfo *pOSyncPluginInfo, OSyncContext *pOSyncContext) |
Connect to sink. | |
static void | mozilla_sync_disconnect (void *data, OSyncPluginInfo *pOSyncPluginInfo, OSyncContext *pOSyncContext) |
Disconnect from sink. | |
static osync_bool | mozilla_sync_discover (void *data, OSyncPluginInfo *pOSyncPluginInfo, OSyncError **ppOSyncError) |
Discover sinks. | |
static void | mozilla_sync_done (void *data, OSyncPluginInfo *pOSyncPluginInfo, OSyncContext *pOSyncContext) |
Called when sync is complete. | |
static void | mozilla_sync_finalize (void *data) |
finalize use of the mozilla-sync plugin | |
static void | mozilla_sync_get_changes (void *data, OSyncPluginInfo *pOSyncPluginInfo, OSyncContext *pOSyncContext) |
Get changes since last sync (or all changes on slow sync). | |
static void * | mozilla_sync_initialize (OSyncPlugin *pOSyncPlugin, OSyncPluginInfo *pOSyncPluginInfo, OSyncError **ppOSyncError) |
initialize use of the mozilla-sync plugin | |
gboolean | mozilla_sync_report_addressbook_changes_to_hash (OSyncMozillaDatabase *pOSyncMozillaDatabase, OSyncPluginInfo *pOSyncPluginInfo, OSyncContext *pOSyncContext) |
Report address book changes since last sync into OSyncMozillaDatabase->OSyncHashTable. | |
gboolean | mozilla_sync_report_calendar_changes_to_hash (OSyncMozillaDatabase *pOSyncMozillaDatabase, OSyncPluginInfo *pOSyncPluginInfo, OSyncContext *pOSyncContext) |
Report calendar changes since last sync into OSyncMozillaDatabase->OSyncHashTable. |
Define Documentation
#define ERR_PRINTF | ( | args... | ) | fprintf(stderr, args); |
Log a message using the pLogFunction
passed
#define GFREE | ( | p | ) | { if (p) g_free(p); } |
#define LOG | ( | level, | |||
message... | ) |
Value:
{ \ if (level<=LOGLEVEL) { \ char *__szMsg=g_strdup_printf(message); \ osync_trace(TRACE_INTERNAL, "%s(%d)%s: %s", basename(__FILE__), __LINE__, __func__, __szMsg); \ g_free(__szMsg); } }
#define LOG_ENTRY | ( | level, | |||
message... | ) |
Value:
{ \ if (level<=LOGLEVEL) { \ char *__szMsg=g_strdup_printf(message); \ osync_trace(TRACE_ENTRY, "%s(%d)%s: %s", basename(__FILE__), __LINE__, __func__, __szMsg); \ g_free(__szMsg); } }
Referenced by get_sync_info(), mozilla_sync_commit_addressbook_change(), mozilla_sync_commit_calendar_change(), mozilla_sync_commit_change(), mozilla_sync_connect(), mozilla_sync_disconnect(), mozilla_sync_discover(), mozilla_sync_done(), mozilla_sync_finalize(), mozilla_sync_get_changes(), mozilla_sync_initialize(), mozilla_sync_report_addressbook_changes_to_hash(), and mozilla_sync_report_calendar_changes_to_hash().
#define LOG_EXIT | ( | level, | |||
message... | ) |
Value:
{ \ if (level<=LOGLEVEL) { \ char *__szMsg=g_strdup_printf(message); \ osync_trace(TRACE_EXIT, "%s(%d)%s: %s", basename(__FILE__), __LINE__, __func__, __szMsg); \ g_free(__szMsg); } }
Referenced by get_sync_info(), mozilla_sync_commit_addressbook_change(), mozilla_sync_commit_calendar_change(), mozilla_sync_commit_change(), mozilla_sync_connect(), mozilla_sync_disconnect(), mozilla_sync_discover(), mozilla_sync_done(), mozilla_sync_finalize(), mozilla_sync_get_changes(), mozilla_sync_initialize(), mozilla_sync_report_addressbook_changes_to_hash(), and mozilla_sync_report_calendar_changes_to_hash().
#define LOG_EXIT_ERROR_OSYNC | ( | level, | |||
ppOSyncError | ) |
Value:
{ \ const char *__szOErr=""; \ if (ppOSyncError) __szOErr=osync_error_print_stack(ppOSyncError); \ osync_trace(TRACE_EXIT_ERROR, "%s(%d)%s: %s", basename(__FILE__), __LINE__, __func__, __szOErr); \ ERR_PRINTF("%s(%d)%s: ****** %s\n", basename(__FILE__), __LINE__, __func__, __szOErr); }
#define LOG_EXIT_ERROR_OSYNC_SZ | ( | level, | |||
ppOSyncError, | |||||
message | ) |
Value:
{ \ const char *__szOErr=""; \ if (ppOSyncError) __szOErr=osync_error_print_stack(ppOSyncError); \ osync_trace(TRACE_EXIT_ERROR, "%s(%d)%s: %s: %s", basename(__FILE__), __LINE__, __func__, message, __szOErr); \ ERR_PRINTF("%s(%d)%s: ****** %s\n", basename(__FILE__), __LINE__, __func__, __szOErr); }
Referenced by get_sync_info(), mozilla_sync_connect(), mozilla_sync_discover(), mozilla_sync_done(), and mozilla_sync_initialize().
#define LOG_EXIT_ERROR_SZ | ( | level, | |||
message | ) |
Value:
{ \ osync_trace(TRACE_EXIT_ERROR, "%s(%d)%s: %s", basename(__FILE__), __LINE__, __func__, message); \ osync_error_set(ppOSyncError, OSYNC_ERROR_GENERIC, message); \ ERR_PRINTF("%s(%d)%s: ****** %s\n", basename(__FILE__), __LINE__, __func__, message); }
Referenced by mozilla_sync_initialize().
#define LOG_EXIT_REPORT_ERROR_MSG | ( | level, | |||
context, | |||||
message... | ) |
Value:
{ \ if (level<=LOGLEVEL) { \ char *__szMsg=g_strdup_printf(message); \ ERR_PRINTF("%s(%d)%s: ****** %s\n", basename(__FILE__), __LINE__, __func__, __szMsg); \ OSyncError *__pError = NULL; \ osync_error_set(&__pError, OSYNC_ERROR_GENERIC, __szMsg); \ osync_context_report_osyncerror(context, __pError); \ osync_trace(TRACE_EXIT_ERROR, __szMsg); \ g_free(__szMsg); } }
Referenced by mozilla_sync_commit_change().
#define LOG_EXIT_REPORT_ERROR_OSYNC | ( | level, | |||
context, | |||||
pError | ) |
Value:
{ \ const char *__szOErr=""; \ if (pError) __szOErr=osync_error_print_stack(&pError); \ ERR_PRINTF("%s(%d)%s: ****** %s\n", basename(__FILE__), __LINE__, __func__, __szOErr); \ osync_context_report_osyncerror(context, pError); \ osync_trace(TRACE_EXIT_ERROR, "%s(%d)%s: %s", basename(__FILE__), __LINE__, __func__, __szOErr); }
Referenced by mozilla_sync_get_changes(), mozilla_sync_report_addressbook_changes_to_hash(), and mozilla_sync_report_calendar_changes_to_hash().
#define LOG_EXIT_REPORT_ERROR_SZ | ( | level, | |||
context, | |||||
message | ) |
Value:
{ \ ERR_PRINTF("%s(%d)%s: ****** %s\n", basename(__FILE__), __LINE__, __func__, message); \ OSyncError *__pError = NULL; \ osync_error_set(&__pError, OSYNC_ERROR_GENERIC, message); \ osync_context_report_osyncerror(context, __pError); \ osync_trace(TRACE_EXIT_ERROR, "%s(%d)%s: %s", basename(__FILE__), __LINE__, __func__, message); }
Referenced by mozilla_sync_commit_addressbook_change(), mozilla_sync_commit_calendar_change(), mozilla_sync_commit_change(), mozilla_sync_connect(), mozilla_sync_disconnect(), mozilla_sync_get_changes(), mozilla_sync_report_addressbook_changes_to_hash(), and mozilla_sync_report_calendar_changes_to_hash().
#define LOG_SENSITIVE | ( | level, | |||
message... | ) |
Value:
{ \ if (level<=LOGLEVEL) { \ char *__szMsg=g_strdup_printf(message); \ osync_trace(TRACE_SENSITIVE, "%s(%d)%s: %s", basename(__FILE__), __LINE__, __func__, __szMsg); \ g_free(__szMsg); } }
#define LOGLEVEL 10000 |
Typedef Documentation
Function Documentation
void addCapabilityEvent | ( | void * | voidSz, | |
void * | voidPCapDef | |||
) |
References _CapDef::fError, LOG, _CapDef::pCapabilities, _CapDef::ppOSyncError, and _CapDef::szObject.
Referenced by mozilla_sync_initialize().
void freeMozillaDatabase | ( | void * | voidOSyncMozillaDatabase, | |
void * | notused | |||
) |
osync_bool get_sync_info | ( | OSyncPluginEnv * | pOSyncPluginEnv, | |
OSyncError ** | ppOSyncError | |||
) |
register the mozilla-sync plugin
Register the mozilla-sync plugin with osync_plugin_env_register_plugin. Just filling out the most important elements of OSyncPlugin
- Parameters:
-
pOSyncPluginEnv Plugin environment in which to register the mozilla-sync plugin ppOSyncError Fill out in case of error
References LOG_ENTRY, LOG_EXIT, LOG_EXIT_ERROR_OSYNC_SZ, mozilla_sync_discover(), mozilla_sync_finalize(), and mozilla_sync_initialize().
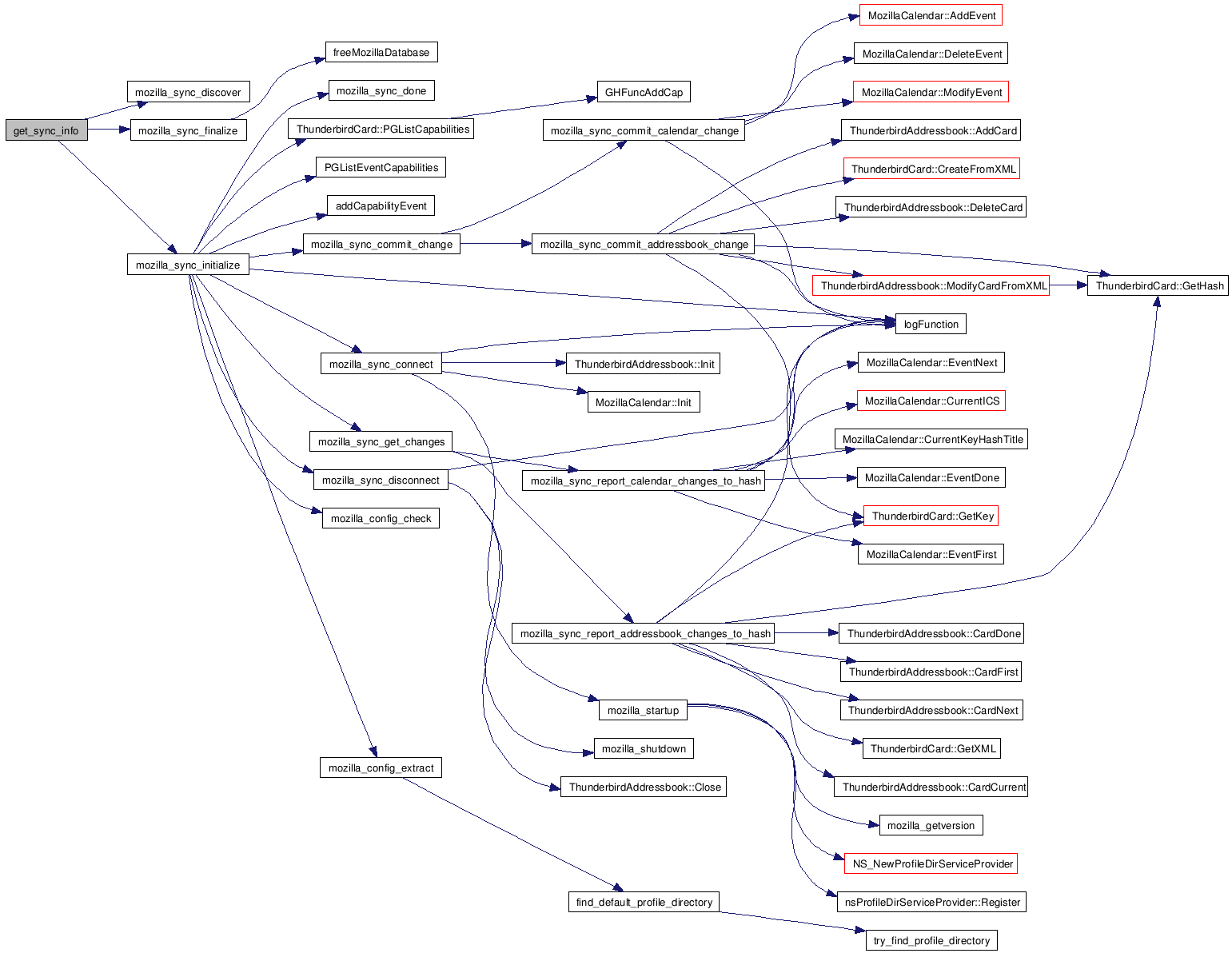
int get_version | ( | void | ) |
void logFunction | ( | int | level, | |
bool | sensitive, | |||
const char * | szFile, | |||
int | iLine, | |||
const char * | szFunc, | |||
const char * | szFormat, | |||
... | ||||
) |
Function to log output from child functions called
- Parameters:
-
level Log level sensitive True if output could contain personal info szFile Name of file we are logging from iLine Line number in szFile szFunc Name of function we are logging from szFormat format specifier and arguments for vprintf
References LOGLEVEL.
static gboolean mozilla_sync_commit_addressbook_change | ( | ThunderbirdAddressbook * | pThunderbirdAddressbook, | |
OSyncChangeType | changeType, | |||
const char * | szKey, | |||
const char * | szXML, | |||
OSyncHashTable * | pHT, | |||
OSyncContext * | pOSyncContext, | |||
OSyncChange * | pChange | |||
) | [static] |
Commit change into thunderbird.
- Parameters:
-
pThunderbirdAddressbook The address book to commit the change into changeType The type of change as reported by OpenSync szKey The key of the mozilla addressbook card szXML The full XML representation of the mozilla addressbook card pHT Pointer to hash table to update pOSyncContext The context of the connect (e.g. to report error) pChange The change we shall commit
References ThunderbirdAddressbook::AddCard(), ThunderbirdCard::CreateFromXML(), ThunderbirdAddressbook::DeleteCard(), ThunderbirdCard::GetHash(), ThunderbirdCard::GetKey(), GFREE, LOG_ENTRY, LOG_EXIT, LOG_EXIT_REPORT_ERROR_SZ, LOG_SENSITIVE, logFunction(), ThunderbirdAddressbook::ModifyCardFromXML(), and ThunderbirdAddressbook::myAddrDatabase.
Referenced by mozilla_sync_commit_change().
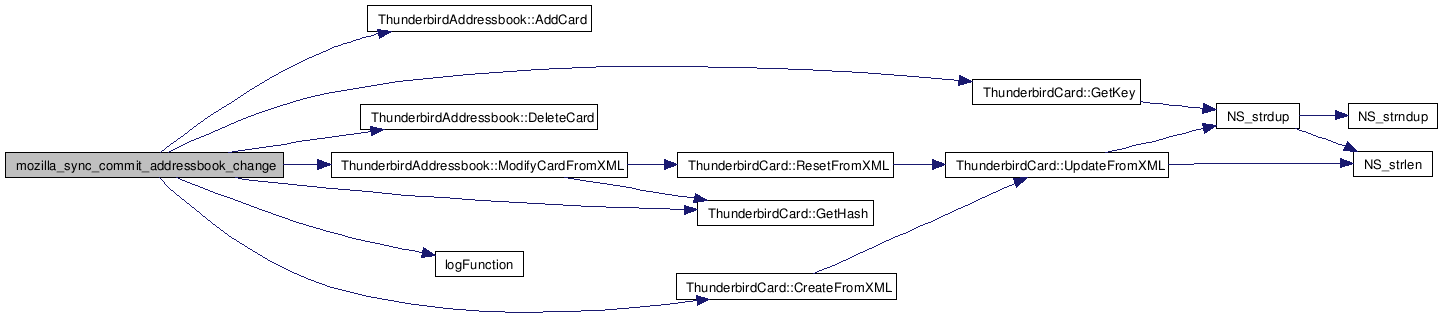
static gboolean mozilla_sync_commit_calendar_change | ( | MozillaCalendar * | pCalendar, | |
OSyncChangeType | changeType, | |||
const char * | szKey, | |||
const char * | szXML, | |||
OSyncHashTable * | pHT, | |||
OSyncContext * | pOSyncContext, | |||
OSyncChange * | pChange | |||
) | [static] |
Commit change into Mozilla calendar.
- Parameters:
-
pCalendar The calendar to commit the change into changeType The type of change as reported by OpenSync szKey The key of the mozilla addressbook card szXML The full XML representation of the mozilla addressbook card pHT Pointer to hash table to update pOSyncContext The context of the connect (e.g. to report error) pChange The change we shall commit
References MozillaCalendar::AddEvent(), MozillaCalendar::DeleteEvent(), GFREE, LOG, LOG_ENTRY, LOG_EXIT, LOG_EXIT_REPORT_ERROR_SZ, LOG_SENSITIVE, logFunction(), and MozillaCalendar::ModifyEvent().
Referenced by mozilla_sync_commit_change().

static void mozilla_sync_commit_change | ( | void * | data, | |
OSyncPluginInfo * | pOSyncPluginInfo, | |||
OSyncContext * | pOSyncContext, | |||
OSyncChange * | pChange | |||
) | [static] |
Commit change.
- Parameters:
-
data The OSyncMozillaEnv pOSyncPluginInfo On entry we have what we gave in get_sync_info. pOSyncContext The context of the connect (e.g. to report error) pChange The change we shall commit
References _OSyncMozillaDatabase::iMozillaDatabaseType, LOG, LOG_ENTRY, LOG_EXIT, LOG_EXIT_REPORT_ERROR_MSG, LOG_EXIT_REPORT_ERROR_SZ, LOG_SENSITIVE, MOZILLA_DATABASE_TYPE_ADDRESSBOOK, MOZILLA_DATABASE_TYPE_CALENDAR, mozilla_sync_commit_addressbook_change(), mozilla_sync_commit_calendar_change(), _OSyncMozillaDatabase::pMozillaCalendar, _OSyncMozillaDatabase::pOSyncHashTable, and _OSyncMozillaDatabase::pThunderbirdAddressbook.
Referenced by mozilla_sync_initialize().
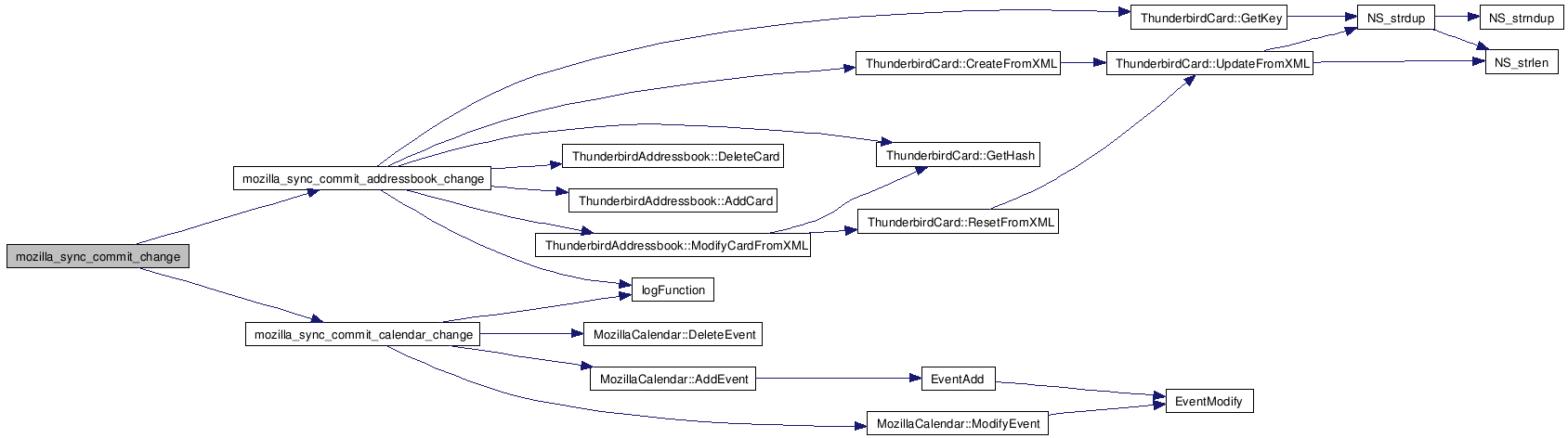
static void mozilla_sync_connect | ( | void * | data, | |
OSyncPluginInfo * | pOSyncPluginInfo, | |||
OSyncContext * | pOSyncContext | |||
) | [static] |
Connect to sink.
- Parameters:
-
data The OSyncMozillaEnv pOSyncPluginInfo On entry we have what we gave in get_sync_info. pOSyncContext The context of the connect (e.g. to report error)
References _OSyncMozillaDatabase::iMozillaDatabaseType, MozillaCalendar::Init(), ThunderbirdAddressbook::Init(), LOG, LOG_ENTRY, LOG_EXIT, LOG_EXIT_ERROR_OSYNC_SZ, LOG_EXIT_REPORT_ERROR_SZ, logFunction(), MOZILLA_DATABASE_TYPE_ADDRESSBOOK, MOZILLA_DATABASE_TYPE_CALENDAR, mozilla_startup(), _OSyncMozillaEnv::mozillaConfig, _OSyncMozillaDatabase::pMozillaCalendar, _OSyncMozillaDatabase::pOSyncHashTable, _OSyncMozillaDatabase::pOSyncObjTypeSink, _OSyncMozillaDatabase::pThunderbirdAddressbook, _MozillaConfig::szAddressbookFile, _OSyncMozillaDatabase::szAnchorKey, _OSyncMozillaDatabase::szAnchorValue, _MozillaConfig::szCalendarId, _MozillaConfig::szProfileDirectory, and _OSyncMozillaEnv::xpcomWasStarted.
Referenced by mozilla_sync_initialize().
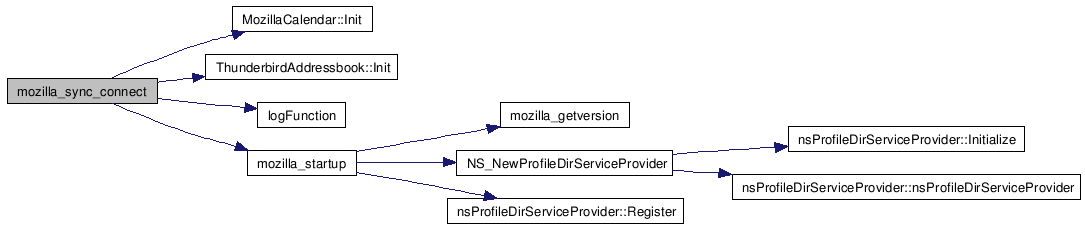
static void mozilla_sync_disconnect | ( | void * | data, | |
OSyncPluginInfo * | pOSyncPluginInfo, | |||
OSyncContext * | pOSyncContext | |||
) | [static] |
Disconnect from sink.
- Parameters:
-
data The OSyncMozillaEnv pOSyncPluginInfo On entry we have what we gave in get_sync_info. pOSyncContext The context of the connect (e.g. to report error)
References ThunderbirdAddressbook::Close(), LOG, LOG_ENTRY, LOG_EXIT, LOG_EXIT_REPORT_ERROR_SZ, logFunction(), mozilla_shutdown(), _OSyncMozillaDatabase::pOSyncHashTable, _OSyncMozillaDatabase::pThunderbirdAddressbook, and _OSyncMozillaEnv::xpcomWasStarted.
Referenced by mozilla_sync_initialize().
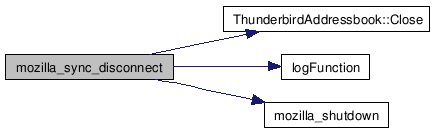
static osync_bool mozilla_sync_discover | ( | void * | data, | |
OSyncPluginInfo * | pOSyncPluginInfo, | |||
OSyncError ** | ppOSyncError | |||
) | [static] |
Discover sinks.
Here we actually tell opensync which sinks are available. For this plugin, we go through the list of databases and enable all, since all have been configured.
- Parameters:
-
data The OSyncMozillaEnv pOSyncPluginInfo On entry we have what we gave in get_sync_info. ppOSyncError Fill out in case of error
References LOG, LOG_ENTRY, LOG_EXIT, LOG_EXIT_ERROR_OSYNC_SZ, _OSyncMozillaEnv::pGListMozillaDatabases, and _OSyncMozillaDatabase::pOSyncObjTypeSink.
Referenced by get_sync_info().
static void mozilla_sync_done | ( | void * | data, | |
OSyncPluginInfo * | pOSyncPluginInfo, | |||
OSyncContext * | pOSyncContext | |||
) | [static] |
Called when sync is complete.
- Parameters:
-
data The OSyncMozillaEnv pOSyncPluginInfo On entry we have what we gave in get_sync_info. pOSyncContext The context of the connect (e.g. to report error)
References LOG, LOG_ENTRY, LOG_EXIT, LOG_EXIT_ERROR_OSYNC_SZ, _OSyncMozillaDatabase::pOSyncHashTable, _OSyncMozillaDatabase::szAnchorKey, and _OSyncMozillaDatabase::szAnchorValue.
Referenced by mozilla_sync_initialize().
static void mozilla_sync_finalize | ( | void * | data | ) | [static] |
finalize use of the mozilla-sync plugin
Simply free the mozilla-sync environment
- Parameters:
-
data The OSyncMozillaEnv
References freeMozillaDatabase(), LOG_ENTRY, LOG_EXIT, and _OSyncMozillaEnv::pGListMozillaDatabases.
Referenced by get_sync_info().

static void mozilla_sync_get_changes | ( | void * | data, | |
OSyncPluginInfo * | pOSyncPluginInfo, | |||
OSyncContext * | pOSyncContext | |||
) | [static] |
Get changes since last sync (or all changes on slow sync).
- Parameters:
-
data The OSyncMozillaEnv pOSyncPluginInfo On entry we have what we gave in get_sync_info. pOSyncContext The context of the connect (e.g. to report error)
References _OSyncMozillaDatabase::iMozillaDatabaseType, LOG, LOG_ENTRY, LOG_EXIT, LOG_EXIT_REPORT_ERROR_OSYNC, LOG_EXIT_REPORT_ERROR_SZ, MOZILLA_DATABASE_TYPE_ADDRESSBOOK, MOZILLA_DATABASE_TYPE_CALENDAR, mozilla_sync_report_addressbook_changes_to_hash(), mozilla_sync_report_calendar_changes_to_hash(), _OSyncMozillaEnv::pOSyncFormatEnv, _OSyncMozillaDatabase::pOSyncHashTable, _OSyncMozillaDatabase::pOSyncMozillaEnv, _OSyncMozillaDatabase::pOSyncObjTypeSink, and _OSyncMozillaDatabase::szObjformat.
Referenced by mozilla_sync_initialize().
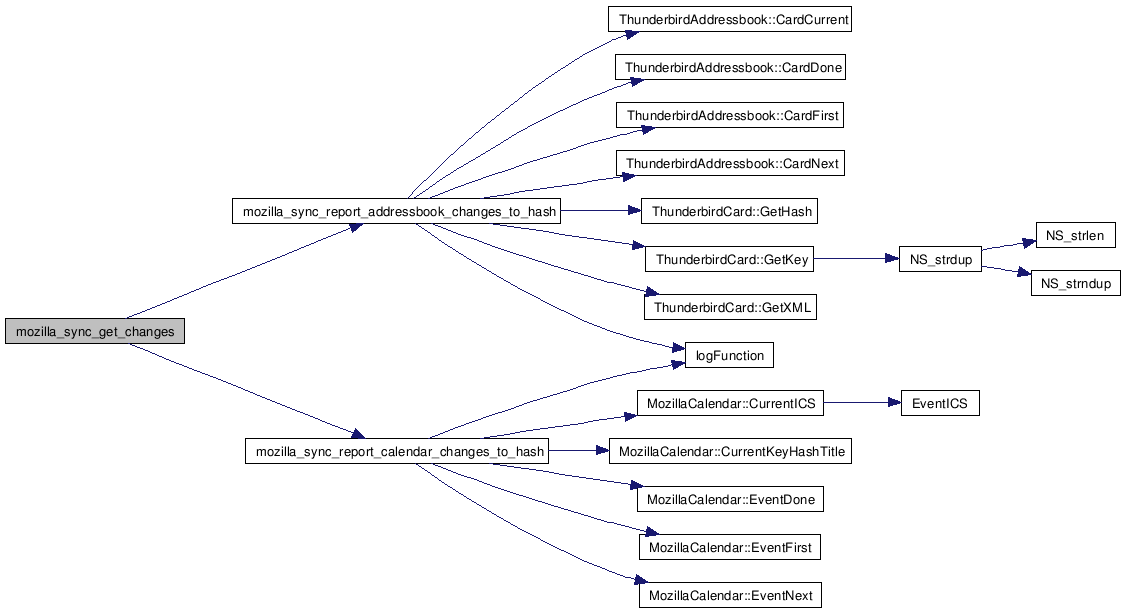
static void* mozilla_sync_initialize | ( | OSyncPlugin * | pOSyncPlugin, | |
OSyncPluginInfo * | pOSyncPluginInfo, | |||
OSyncError ** | ppOSyncError | |||
) | [static] |
initialize use of the mozilla-sync plugin
In initialize, we get and parse the config for the plugin. Here we also must register all _possible_ objtype sinks
- Parameters:
-
pOSyncPlugin Not used pOSyncPluginInfo On entry we have what we gave in get_sync_info. Now TODO ppOSyncError Fill out in case of error
References addCapabilityEvent(), _CapDef::fError, _OSyncMozillaDatabase::iMozillaDatabaseType, LOG, LOG_ENTRY, LOG_EXIT, LOG_EXIT_ERROR_OSYNC_SZ, LOG_EXIT_ERROR_SZ, logFunction(), mozilla_config_check(), mozilla_config_extract(), MOZILLA_DATABASE_TYPE_ADDRESSBOOK, MOZILLA_DATABASE_TYPE_CALENDAR, mozilla_sync_commit_change(), mozilla_sync_connect(), mozilla_sync_disconnect(), mozilla_sync_done(), mozilla_sync_get_changes(), _OSyncMozillaEnv::mozillaConfig, _CapDef::pCapabilities, ThunderbirdCard::PGListCapabilities(), PGListEventCapabilities(), _OSyncMozillaEnv::pGListMozillaDatabases, _OSyncMozillaEnv::pOSyncFormatEnv, _OSyncMozillaDatabase::pOSyncMozillaEnv, _OSyncMozillaDatabase::pOSyncObjTypeSink, _CapDef::ppOSyncError, _MozillaConfig::szAddressbookFile, _OSyncMozillaDatabase::szAnchorKey, _OSyncMozillaDatabase::szAnchorValue, _MozillaConfig::szCalendarId, _CapDef::szObject, _OSyncMozillaDatabase::szObjformat, _OSyncMozillaDatabase::szObjtype, and _MozillaConfig::szProfileDirectory.
Referenced by get_sync_info().
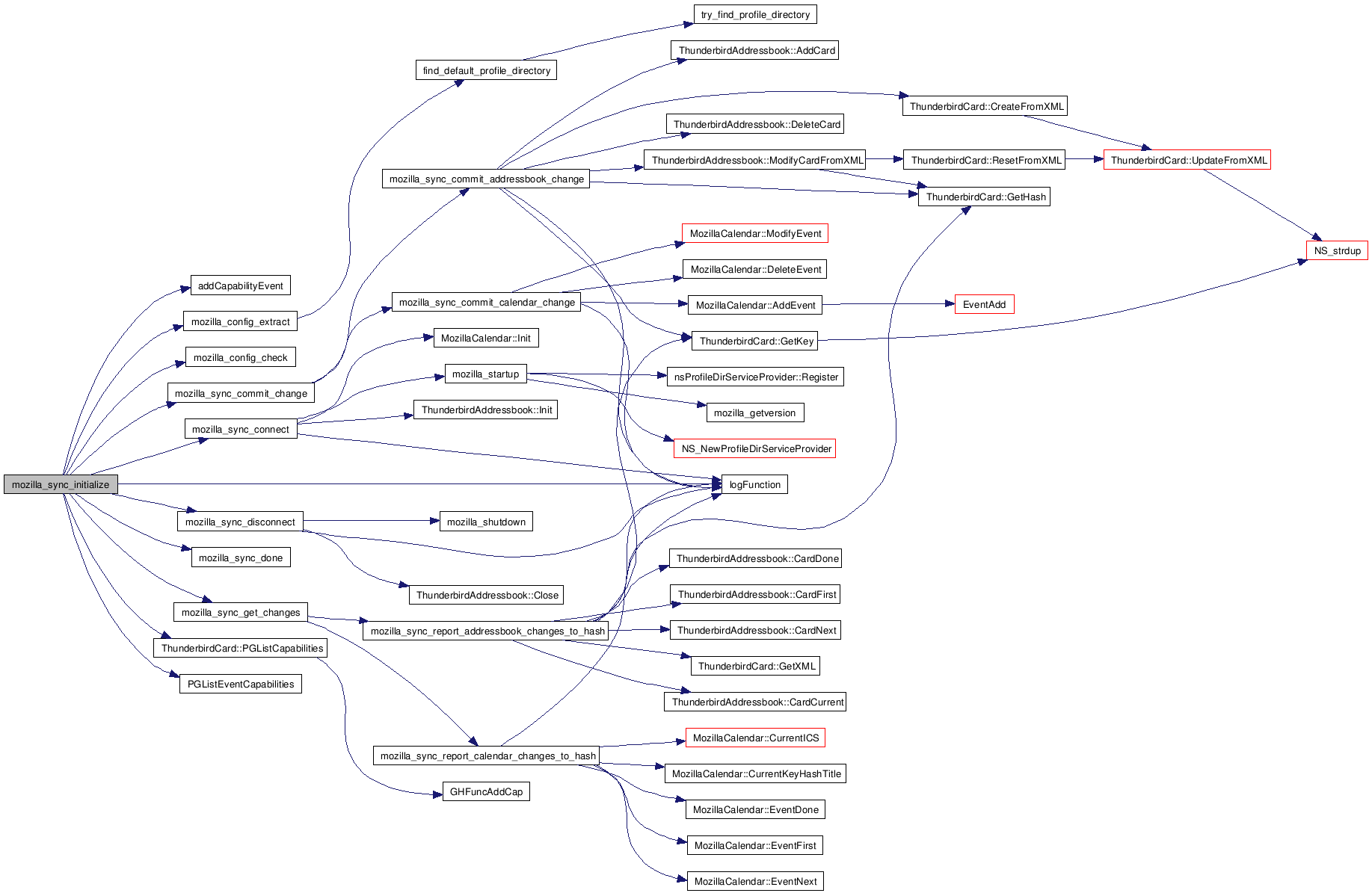
gboolean mozilla_sync_report_addressbook_changes_to_hash | ( | OSyncMozillaDatabase * | pOSyncMozillaDatabase, | |
OSyncPluginInfo * | pOSyncPluginInfo, | |||
OSyncContext * | pOSyncContext | |||
) |
Report address book changes since last sync into OSyncMozillaDatabase->OSyncHashTable.
- Parameters:
-
pOSyncMozillaDatabase The database we have to report on pOSyncPluginInfo On entry we have what we gave in get_sync_info. pOSyncContext The context of the connect (e.g. to report error)
- Returns:
- TRUE on success; FALSE on error
References ThunderbirdAddressbook::CardCurrent(), ThunderbirdAddressbook::CardDone(), ThunderbirdAddressbook::CardFirst(), ThunderbirdAddressbook::CardNext(), ThunderbirdCard::GetHash(), ThunderbirdCard::GetKey(), ThunderbirdCard::GetXML(), GFREE, _OSyncMozillaDatabase::iMozillaDatabaseType, LOG, LOG_ENTRY, LOG_EXIT, LOG_EXIT_REPORT_ERROR_OSYNC, LOG_EXIT_REPORT_ERROR_SZ, LOG_SENSITIVE, logFunction(), MOZILLA_DATABASE_TYPE_ADDRESSBOOK, ThunderbirdAddressbook::myAddrDatabase, _OSyncMozillaEnv::pOSyncFormatEnv, _OSyncMozillaDatabase::pOSyncHashTable, _OSyncMozillaDatabase::pOSyncMozillaEnv, _OSyncMozillaDatabase::pOSyncObjTypeSink, _OSyncMozillaDatabase::pThunderbirdAddressbook, and _OSyncMozillaDatabase::szObjformat.
Referenced by mozilla_sync_get_changes().
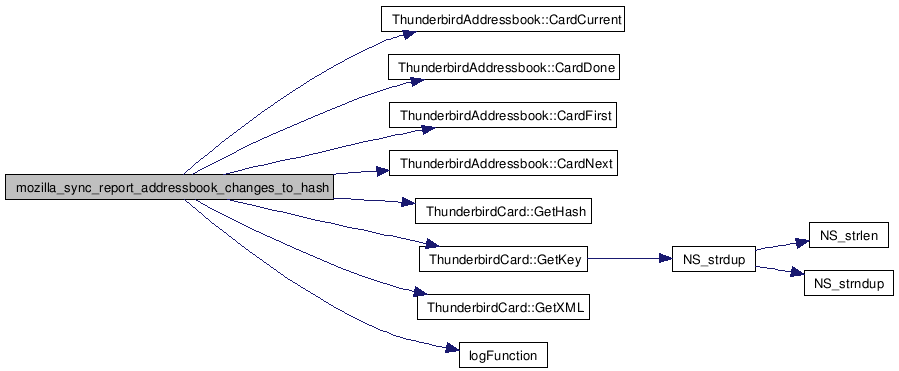
gboolean mozilla_sync_report_calendar_changes_to_hash | ( | OSyncMozillaDatabase * | pOSyncMozillaDatabase, | |
OSyncPluginInfo * | pOSyncPluginInfo, | |||
OSyncContext * | pOSyncContext | |||
) |
Report calendar changes since last sync into OSyncMozillaDatabase->OSyncHashTable.
- Todo:
- mozilla_sync_report_calendar_changes_to_hash and mozilla_sync_report_addressbook_changes_to_hash are quite similar. Could we merge them?
- Parameters:
-
pOSyncMozillaDatabase The database we have to report on pOSyncPluginInfo On entry we have what we gave in get_sync_info. pOSyncContext The context of the connect (e.g. to report error)
- Returns:
- TRUE on success; FALSE on error
References MozillaCalendar::CurrentICS(), MozillaCalendar::CurrentKeyHashTitle(), MozillaCalendar::EventDone(), MozillaCalendar::EventFirst(), MozillaCalendar::EventNext(), _OSyncMozillaDatabase::iMozillaDatabaseType, LOG, LOG_ENTRY, LOG_EXIT, LOG_EXIT_REPORT_ERROR_OSYNC, LOG_EXIT_REPORT_ERROR_SZ, LOG_SENSITIVE, logFunction(), MOZILLA_DATABASE_TYPE_CALENDAR, _OSyncMozillaDatabase::pMozillaCalendar, _OSyncMozillaEnv::pOSyncFormatEnv, _OSyncMozillaDatabase::pOSyncHashTable, _OSyncMozillaDatabase::pOSyncMozillaEnv, _OSyncMozillaDatabase::pOSyncObjTypeSink, and _OSyncMozillaDatabase::szObjformat.
Referenced by mozilla_sync_get_changes().
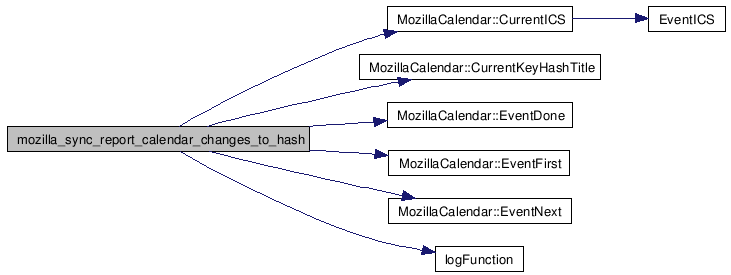